Key Takeaways:
- Abstraction and modularity simplify complex data and dividing problems into smaller modules enhances code readability, reusability, and maintainability.
- Continuous improvement through refactoring and prioritizing simplicity in code design leads to easier maintenance, testing, and understanding.
- SOLID five principles (SRP, OCP, LSP, ISP, DIP) promote a modular, extensible, and maintainable software architecture.
The principles of software development are guiding rules that empower software developers to handle the intricate processes to ensure effectiveness. With these principles in mind, a software developer team minimizes the effort and time required to execute software systems. Furthermore, it diminishes the chances of errors during the design process, which takes the overall efficiency a step forward.
In this blog, we have gathered the top 10 key principles of software development and divided them into two major categories: basic and SOLID software development principles.
Let’s delve deep into these principles.
Basic Software Development Principles
The following list of principles constitutes the basics of any software development project. They are the essential ingredients to get the desired results at the end of the software development cycle. Moreover, these coding principles build the mindset needed for the efficient completion of software. Here, we have included the following principles:
1. Data Abstraction
In software development, the term abstraction means simplification of data, which is a key concept in both front-end and back-end web development. In this process, software developers convert the large data sets into smaller ones that are more understandable and manageable. Abstraction can fundamentally boost productivity and enhance the quality of software development.
An American computer scientist, John V. Guttag, described abstraction in the following words, “ The essence of abstraction is preserving information that is relevant in a given context and forgetting information that is irrelevant in that context.”
The above statement sums up the role of abstraction in software development. You simply hide the complex code and extra information with abstraction. The benefits of data abstraction in software development include easier code management, facilitating testing, and enhanced scalability.
2. Modularity
Modularity is a design approach in software development. Its purpose is to divide large and complex problems into smaller ones. It has multiple advantages while coding for large software.
First, it makes code easier to write and understand. Second, by naming each module separately, you can integrate each part later on to test a complete functional software. Third, modularity makes code reusable. It means you can use the older code while building newer software to mimic a similar function.
Modularity operates on two key principles. Cohesion and coupling. First, cohesion involves the relation of elements within a module; higher cohesion is good, meaning a purpose-oriented module. Second, coupling refers to the interdependence of modules. Low coupling is desirable because it allows the modification of modules due to their minimal involvement in each other’s functions.
3. Refactoring
Refactoring is defined as the process of restructuring code with no changes in the original software structure. Its main purpose is to enhance the quality of internal code while keeping the code’s external behavior intact.
Refactoring has multiple benefits, including improvement in code readability, minimum complexities,exploring the leading AI frameworks and identification of hidden vulnerabilities in the software similar to exploring the bug life cycle process. The rationale behind the refactoring process is that small changes to code produce a cumulative effect while preserving the software’s originality.
Martin Fowler made a list of best practices for refactoring in the software development process in his book Refactoring: Improving the Design of Existing Code. These practices include planning, setting clear objectives, frequent testing, automation, and regular updates post-refactoring.
4. KISS (Keep it Simple Stupid) Principle
According to this software development principle, products, designs, systems, and solutions work best if they’re kept simple. Simplicity of code should be our top priority, and complexity should be avoided.
Why should the KISS principle be followed in code? First, code becomes easier to maintain and change. Second, it also becomes easy to understand, and third, it is easy to test. Now, let’s take a look at how to apply KISS in our software development projects.
The first step is to write smaller, readable, and transparent code. Implement modular programming to divide the large and complex code into smaller versions. Moreover, code in a way that helps you reuse the code whenever required.
5. Problem Partitioning
Problem partitioning involves breaking up computation tasks into smaller parts. In this way, less time is spent on computation. For parallel algorithms, partitioning performance is ideal. The main challenge comes with the inability to merge these results into smaller parts to arrive at an outcome.
The benefits of problem partitioning include the following: software becomes easy to understand, test, maintain, and expand. There is also an added advantage of outsourcing your project and building remote teams to augment the process of software development.
It is important to understand that division into smaller parts does not mean separation of roles. These smaller parts will always work in collaboration. Once these parts are together, then they complete the system and perform ideally.
SOLID Software Development Principles
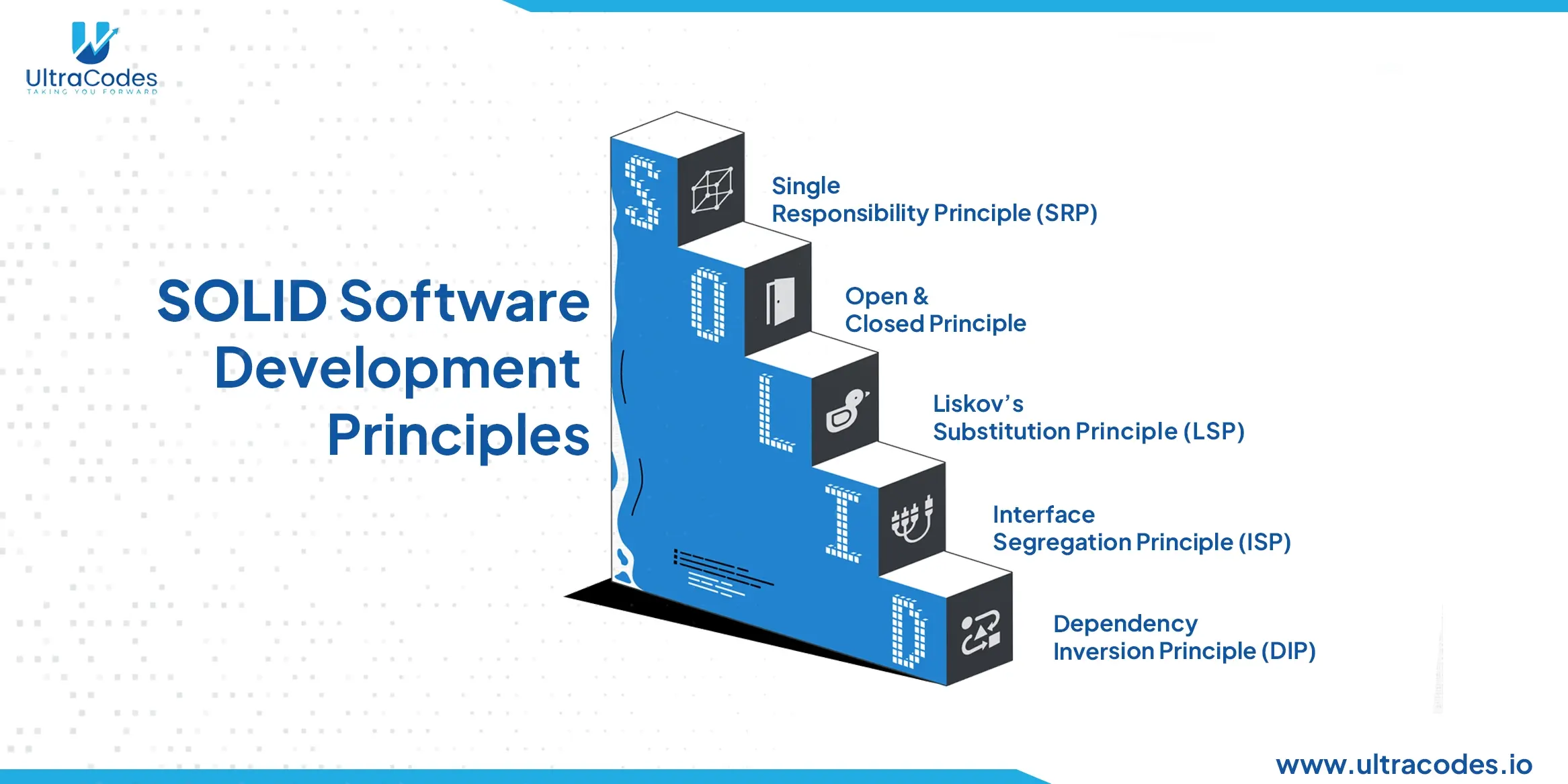
Robert C. Martin was the man behind the SOLID principle. He was also referred to as Uncle Bob, and his proposed principles are a standard in programming. Following are the acronyms of the five principles:
- Single Responsibility Principle (SRP)
- Open/Closed Principle
- Liskov’s Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
6. Single Responsibility Principle (SRP)
The single responsibility principle states that every class should do one job within a software development cycle. Multiple benefits are attached to this approach, such as the application of expertise in respective areas and the boost in work effectiveness and efficiency.
Let’s try to understand this principle using an example.
A car mechanic is responsible for repairing and maintaining vehicles. His tasks involve diagnosing problems, maintenance, and replacing parts.
However, the single responsibility principle will be violated if the mechanic is also involved in selling cars, handling payments, and managing appointments. Therefore, in order to adhere to SRP, the garage should have different people working on other tasks.
7. Open/Closed Principle
According to the Open/Closed principle, class behavior should be able to extend but not modify. Software entities should be open for extension but closed for modifications.
Here’s an example to explain the Open/Closed Principle:
Imagine a company has a reporting tool that generates various types of reports. Initially, the reporting tool only supports outputting reports in PDF format. Now, the company wants to add the ability to export reports in other formats like CSV or Excel.
To adhere to the OCP, the company could design the reporting tool with extensibility in mind. This can be achieved by:
Create an interface (e.g., ReportFormatter) that defines a standard way to format reports. Develop separate classes for each format, each implementing the ReportFormatter interface. For example:
- PDFReportFormatter
- CSVReportFormatter
- ExcelReportFormatter
The reporting tool’s core logic interacts with the formatters through the ReportFormatter interface. When a user selects a format, the tool uses the appropriate formatter to generate the report.
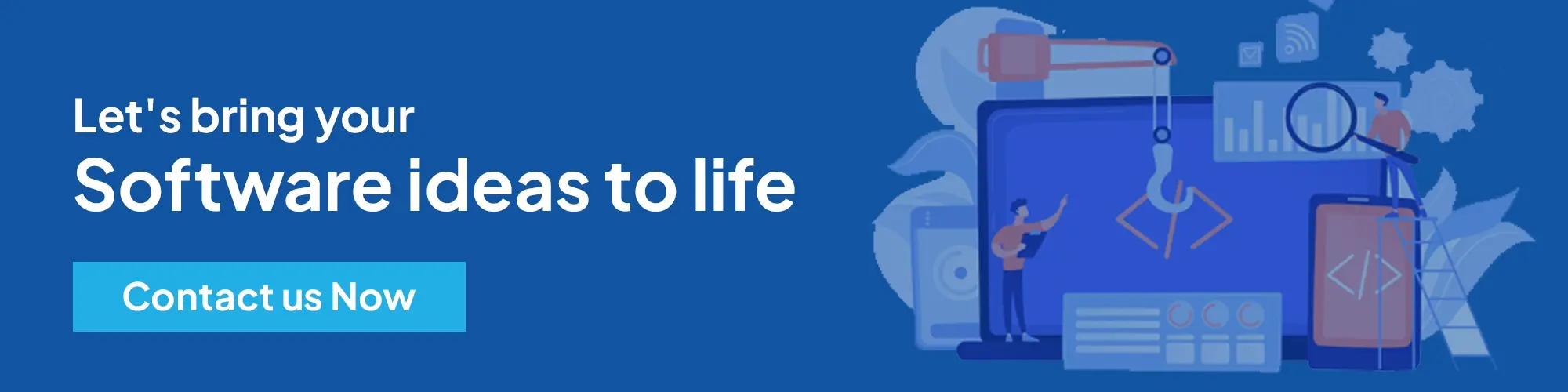
8. Liskov’s Substitution Principle (LSP)
Barbara Liskov came up with this principle in 1987, and principle states that “Derived or child classes must be substitutable for their base or parent classes.” With the application of this principle, any child class should be able to replace the parent class without any deviations.
Let’s explore this complex concept with an example:
Imagine you have a class Bird with a method fly(). This class is meant to represent birds in general, and the fly() method encapsulates the behavior of birds taking flight.
Now, you create a subclass Ostrich to represent ostriches. Ostriches are birds, but they cannot fly.
If you simply inherit the fly()method from the Bird class into the Ostrich class without modifying it, you violate the LSP. Why? If you have a piece of code that expects a Bird object and calls its fly() method, it would reasonably assume that the bird can fly. If you pass an Ostrich object instead, the code will break, as ostriches cannot fly.
9. Interface Segregation Principle (ISP)
Similar to the single responsibility principle, ISP is the first principle applicable to interfaces and not to the classes as part of SOLID. According to this principle, each interface must be relevant to it. In this case, avoiding fat interfaces is necessary, and focusing on small client-specific interfaces is paramount.
Here’s an example to exhibit this principle:
Imagine a modern multi-function printer that can perform various tasks like printing, scanning, photocopying, and faxing. Now, consider the software interface used to control this printer.
To follow the ISP, the printer’s interface should be segregated into smaller, more focused interfaces:
- PrinterInterface: Contains methods only related to printing (e.g., printDocument(), printSettings()).
- ScannerInterface: Contains methods for scanning documents (e.g., scanDocument(), scanSettings()).
10. Dependency Inversion Principle (DIP)
DIP states that “high-level modules should not depend on low-level modules; both should rely on abstractions.” Moreover, abstractions should be free from details, which in turn should depend on abstractions.
Let’s delve deep into the dependency inversion principle with an example:
Imagine a coffee shop that uses various types of coffee machines – drip coffee makers, espresso machines, and cappuccino machines. To apply the DIP, the coffee shop could introduce an abstraction layer:
Create an interface called CoffeeMachine that defines common operations like brewCoffee(), frothMilk(), etc. Each coffee machine model (drip, espresso, cappuccino) would have its class that implements the CoffeeMachine interface, providing specific implementations for the common operations. The coffee shop’s software system would interact with coffee machines only through the CoffeeMachine interface, not the concrete classes.
Practical Use Cases of Software Development Principles
Microsoft (Open/Closed Principle)
Microsoft’s .NET framework is a prime example of the Open/Closed Principle in action. The framework is designed to allow developers to create custom components that seamlessly integrate with the existing system.
- Benefits: This approach enables Microsoft to maintain a stable core framework while empowering developers to add new functionalities without modifying the base code.
Amazon (Dependency Inversion Principle)
Amazon Web Services (AWS) is a cloud computing platform that embodies the Dependency Inversion Principle. AWS provides a vast array of services (storage, computing, databases, etc.) that interact through well-defined interfaces.
- Benefits: This decoupling allows Amazon to evolve and improve individual services independently without impacting the overall system. Developers can choose the specific AWS services they need and easily switch between them as requirements change.
Conclusion: Mastering the Principles of Software Development
Mastering the principles of software development is a necessity for software developers in modern times. In the discussion above, the 10 software development principles, divided into basic and SOLID categories, offer a comprehensive guide to creating efficient, maintainable, and scalable software.
By adhering to these principles, developers can streamline the development process, reduce errors, and build software that can easily adapt to future changes. Furthermore, by understanding and applying these solid software development principles, developers can elevate the quality of their software projects and deliver solutions that meet both current and future needs.
